-
Posts for year 2014, total posts 59 / 59 migrated
All Posts for 2014
My Site - 2014 in review
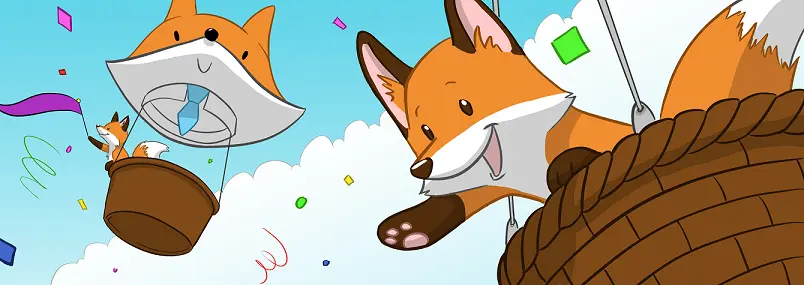
The WordPress.com stats helper monkeys prepared a 2014 annual report for this blog.
Continue Reading...Unholy Matrimony - wGet and PowerShell together
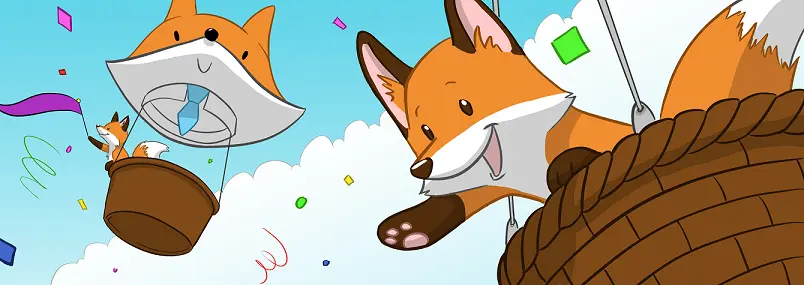
XML has been around for a LONG time. But just like working with HTML, it still kind of stinks. If you want to reach into a file and pull out values within certain tags, you’d better become a pro with Xpath or be prepared to create some REALLY ugly Regex. For instance, if we wanted to grab the values within the tag we care about for this blog post, the regex would be this simple little number.
Continue Reading...PowerShell Version 5, What's new!
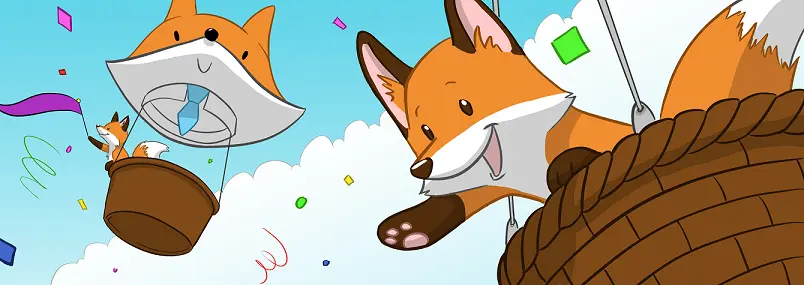
PowerShell native switch configuration
Continue Reading...Solving the DSC Pull Chicken and Egg problem

Continue Reading...
Working with Web Services, SOAP, PHP and all the REST with PowerShell
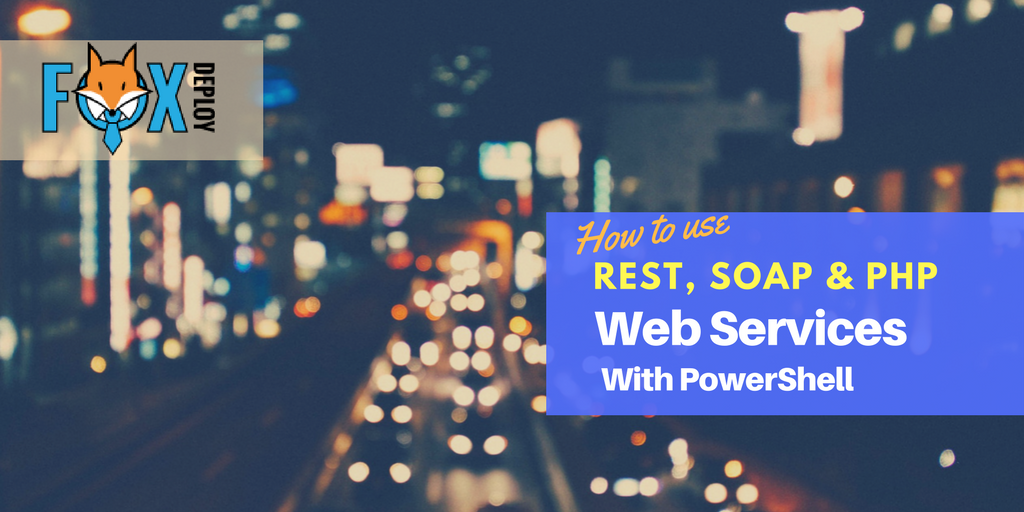
Previous
Page: of
Next