Quicky: How to use Server Nano TP4 in Hyper-V
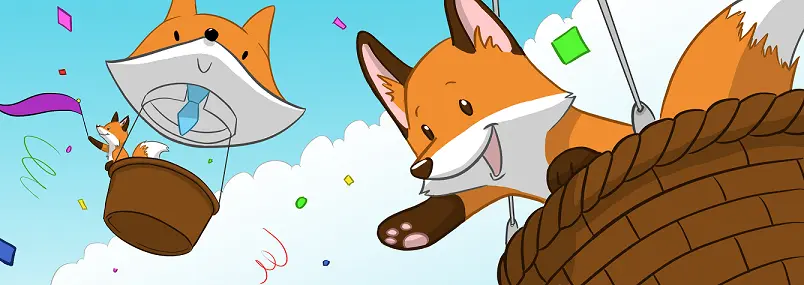
Hey guys,
Continue Reading...Solved: Cisco AnyConnect 'Session Ended' error
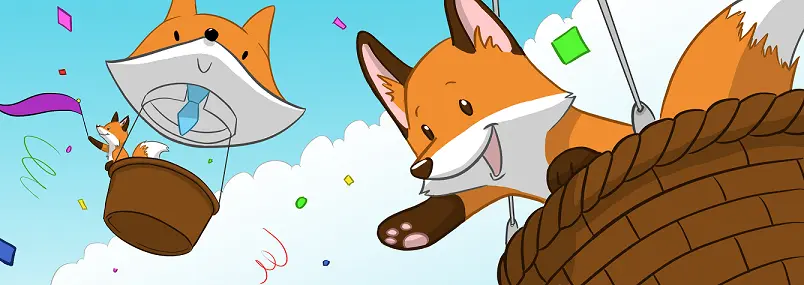
This was kicking my butt today, but turns out that it had an easy work around.
Continue Reading...Listen to me on Coding101!
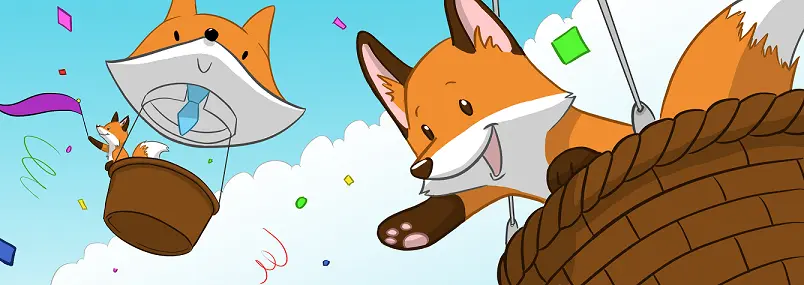
Last week, I had an amazing time at the Microsoft MVP Summit, it was a dream come true! Â Speaking of true, I even got to meet Jim Truher and Bruce Payette! Â Simply a wonderful, wonderful time.
Continue Reading...Using PowerShell and oAuth
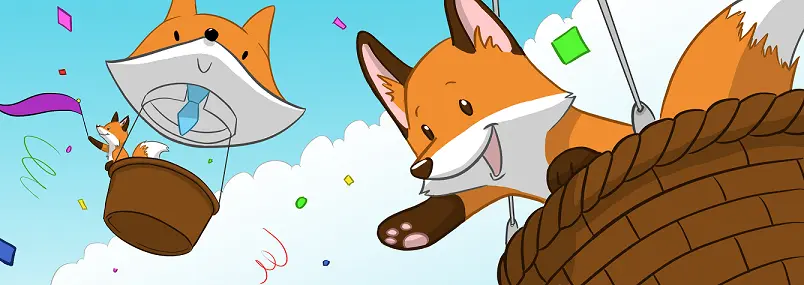
Windows vs Intel Raid Performance Smackdown
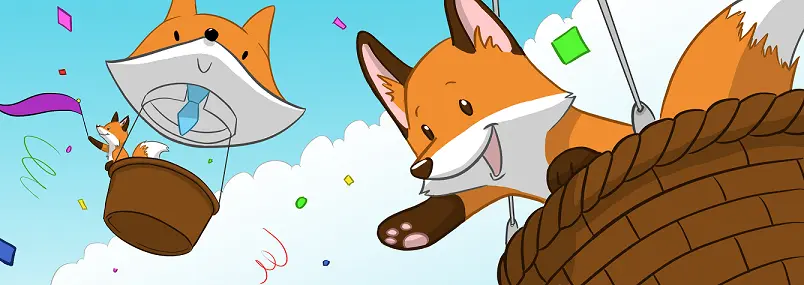
Update: Turns out I was pretty wrong about Storage Spaces and have been totally schooled and corrected about them here, on Reddit and at Redmond.  I’ll be revising this post heavily, adding some iometer numbers.  Stay tuned!
Continue Reading...Hyper-V on Windows 10, how to fix a broken VHD
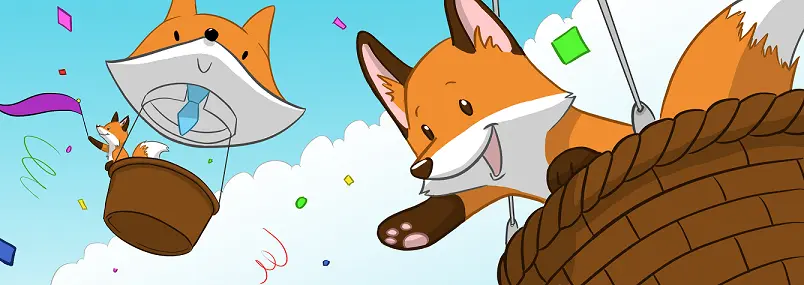
I’m running Windows 10 w/ Hyper-V on my home lab pc, and found a strange Hyper-V error this week.
Continue Reading...