Hyper-V on Windows 10, how to fix a broken VHD
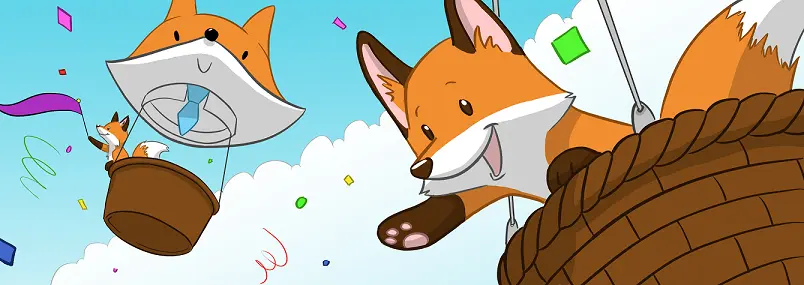
I’m running Windows 10 w/ Hyper-V on my home lab pc, and found a strange Hyper-V error this week.
Continue Reading...Part IV - PowerShell GUIs - how to handle events and create a Tabbed Interface
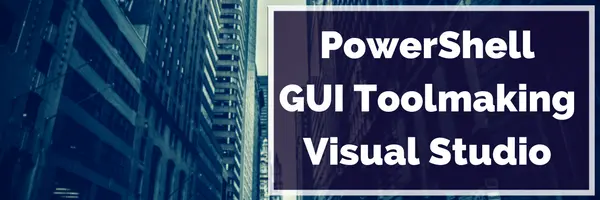
Continue Reading...
'GUI your life with PowerShell' - Slides and Scripts available
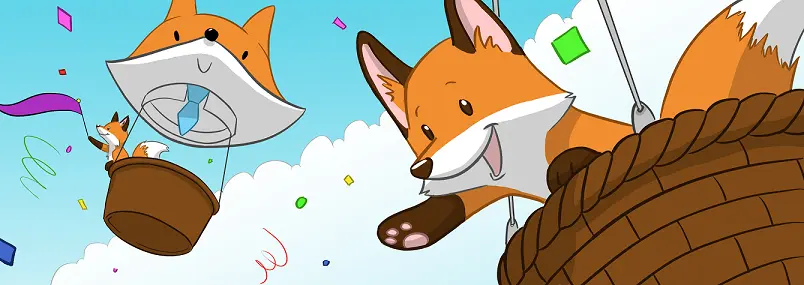
Here are the slides and scripts from my session at Atlanta TechStravaganza at the Georgia Tech Research Institute.
Continue Reading...New in Windows 10: Changing home to Pro w/ no reinstall!

One of the hotly anticipated features of Windows 10 is the ability to change your Windows version on the fly, without even needing to reinstall the OS!
Continue Reading...Using PowerShell to find drivers for Device Manager
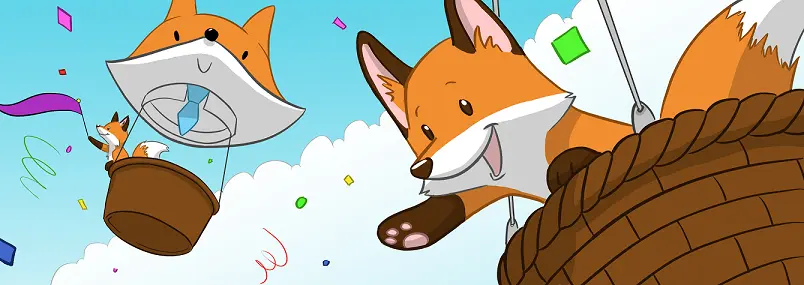
One of the most arduous tasks for a ConfigMgr admin is to build images to support new models of hardware for Operating System Distribution. As a SCCM Engineer, I’ve done this process for probably more than a hundred models now, and here’s how it normally goes.
Continue Reading...Recovering your Dedeuped Files on Windows 10
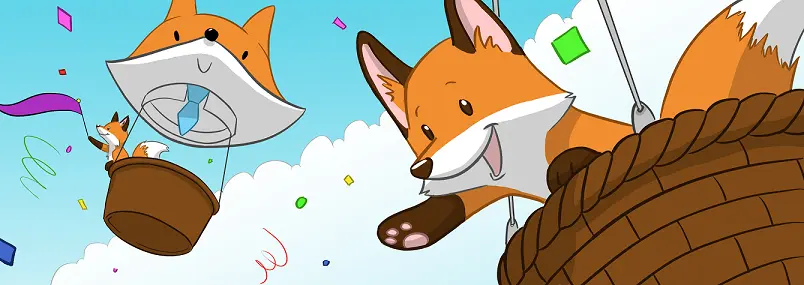
Were you one of those who installed the server 2012 binaries into your Windows 8.1 to enable Disk Deduplication? Did you turn on dedupe on all of your drives, saving hundreds of gigs of storage space, then upgrade to Windows 10?
Continue Reading...