Solving the DSC Pull Chicken and Egg problem

Continue Reading...
Working with Web Services, SOAP, PHP and all the REST with PowerShell
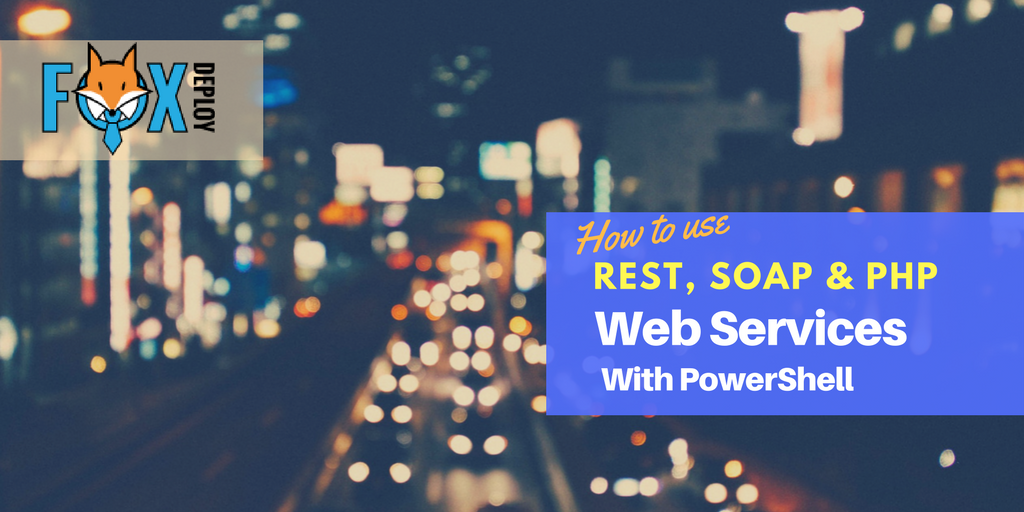
Question Time: when I want a property, PowerShell gives the whole object!
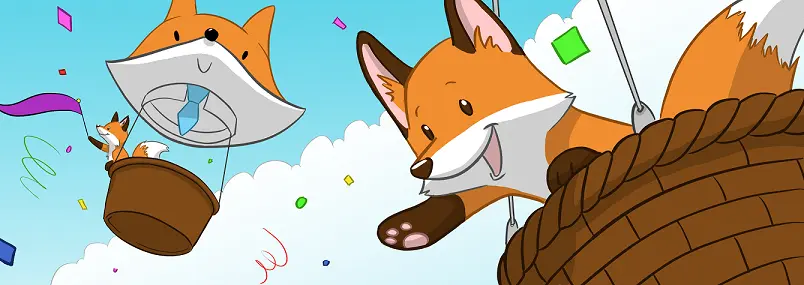
I’m posting today on a topic I see over and over again in the forums, on reddit, and have run into myself numerous times. Every person I’ve ever taught PowerShell runs into it too, and most authors have covered this at some point, including Don Jones in ‘The big book of PowerShell Gotchas’.
Continue Reading...The Five Commandments of managing and recovering from a serious outage (with your job!)
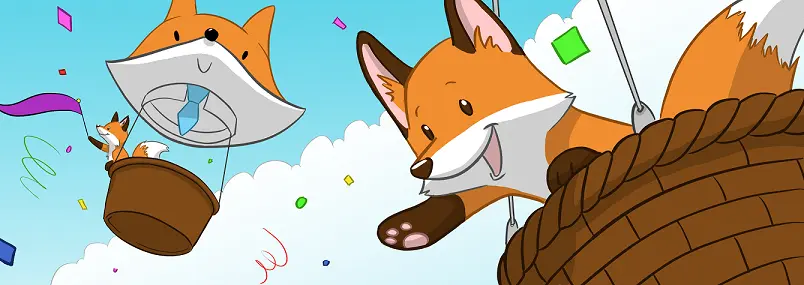
Topic introduction: Consulting 101
Continue Reading...Set PowerShell as your CLI of choice in Windows 8. WITH POWERSHELL
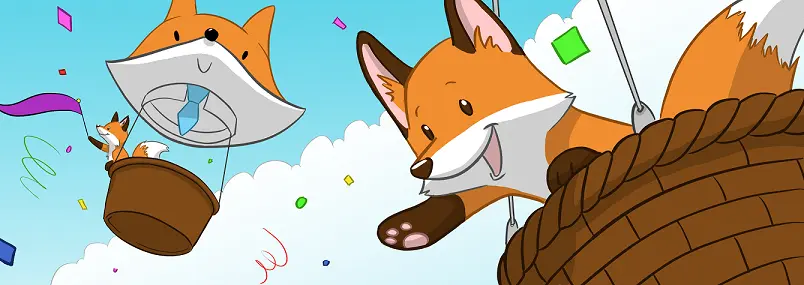
In a conversation on Twitter, I lamented with some friends that Hitting Windows+X in Windows 8 and above opens up the admin window, but still–In 2014–lists Command Prompt as the CLI.
Continue Reading...PushBullet + PowerShell = PowerBullet!
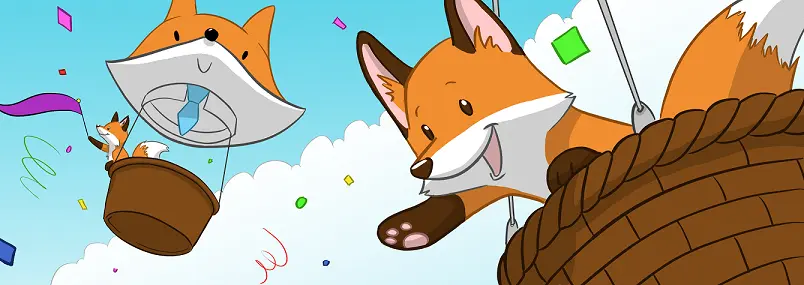
A friend and colleague of mine, Chris Townsend were talking over lunch recently, when he lamented that during a very long scripted process, he would have to manually check back in for status checks to see how this process was going, and might waste a whole weekend in front of the keyboard. Â We began trying to think of ways to get notifications if he needed to intervene and manually do something.
Continue Reading...